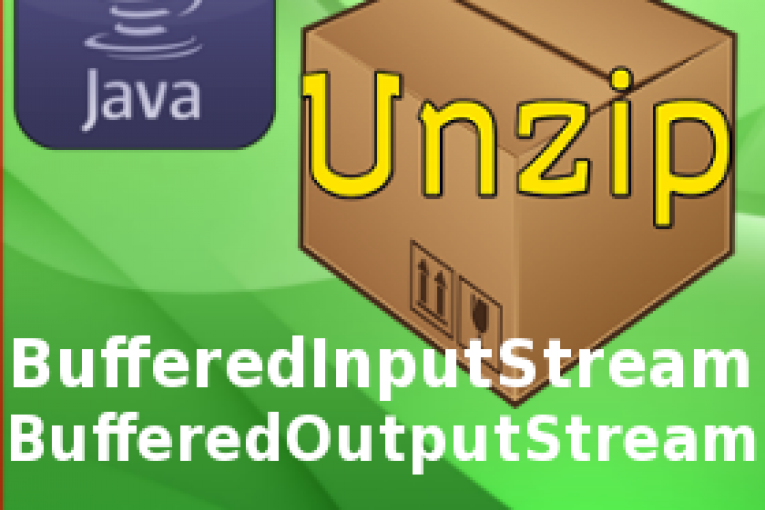
Riporto una classe molto utile per effettuare estrazioni di archivi in formato ZIP e GZIP compressi su algoritmo deflate. L’operazione di estrazione usa l’utility java.util.zip presente all’interno della libreria nativa rt.jar di JDK. Per aumentare le performance sulle operazioni di I/O sui file, vengono utilizzati gli oggetti java.io.BufferedInputStream e java.io.BufferedOutputStream.
package eu.giuseppeurso.utils; import java.io.BufferedInputStream; import java.io.BufferedOutputStream; import java.io.File; import java.io.FileOutputStream; import java.io.IOException; import java.util.Enumeration; import java.util.zip.ZipEntry; import java.util.zip.ZipFile; public class ZipUtils { public static void unzip(String strZipFile) { try { File fSourceZip = new File(strZipFile); String zipPath = strZipFile.substring(0, strZipFile.length() - 4); File temp = new File(zipPath); temp.mkdir(); System.out.println(zipPath + " created"); ZipFile zipFile = new ZipFile(fSourceZip); Enumeration<?> e = zipFile.entries(); while (e.hasMoreElements()) { ZipEntry entry = (ZipEntry) e.nextElement(); File destinationFilePath = new File(zipPath, entry.getName()); destinationFilePath.getParentFile().mkdirs(); if (entry.isDirectory()) { continue; } else { System.out.println("Extracting " + destinationFilePath); BufferedInputStream bis = new BufferedInputStream(zipFile.getInputStream(entry)); int b; byte buffer[] = new byte[1024]; FileOutputStream fos = new FileOutputStream(destinationFilePath); BufferedOutputStream bos = new BufferedOutputStream(fos,1024); while ((b = bis.read(buffer, 0, 1024)) != -1) { bos.write(buffer, 0, b); } // flush the output stream and close it. bos.flush(); bos.close(); // close the input stream. bis.close(); } } } catch (IOException ioe) { System.out.println("IOError :" + ioe); } } }